Introduction:
Unit testing is a crucial aspect of software development, ensuring that individual components of your application work as expected. Writing effective unit tests helps catch bugs early, facilitates refactoring, and ensures your codebase remains robust and maintainable. In this tutorial, we’ll explore how to write effective unit tests in JavaScript using popular testing frameworks like Jest and Mocha.
1. Setting Up Your Testing Environment
Before diving into writing tests, it’s essential to set up your testing environment. We’ll use Jest in this tutorial due to its simplicity and popularity.
1.1 Install Jest: First, you’ll need to install Jest as a development dependency in your project.

1.2 Configure Jest: Next, add a test script to your package.json
file to run Jest.
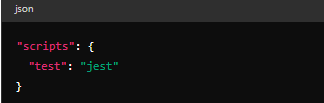
2. Writing Your First Unit Test
Unit tests are written to test the smallest parts of an application, typically individual functions or methods. Let’s start by writing a simple function and a test for it.
2.1 Create a Function:
Create a file math.js
with the following code:
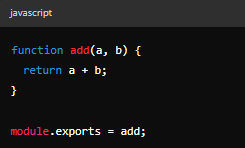
2.2 Write a Test:
Create a test file math.test.js
:
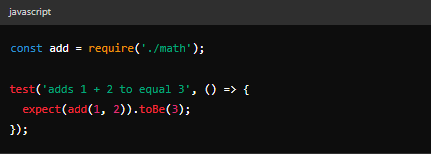
2.3 Run Your Test:
Run the test using the command:
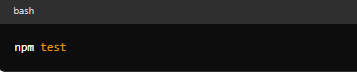
You should see an output indicating that the test passed.
3. Structuring Your Tests
Organize your tests to make them readable and maintainable.
3.1 Describe Blocks:
Use describe
blocks to group related tests. This helps in organizing tests logically.
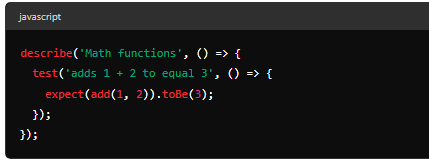
3.2 Setup and Teardown:
Use beforeEach
, afterEach
, beforeAll
, and afterAll
for setup and teardown logic
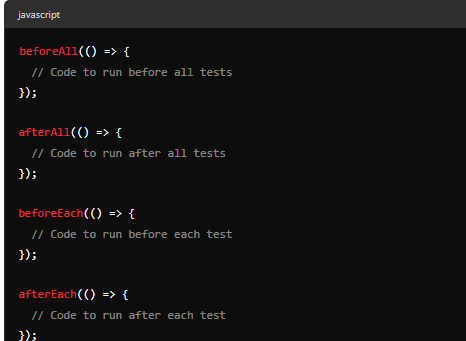
4. Writing More Complex Tests
Let’s add a new function and write tests for more complex scenarios.
4.1 Create a Subtract Function:
Add a subtract function to math.js
:
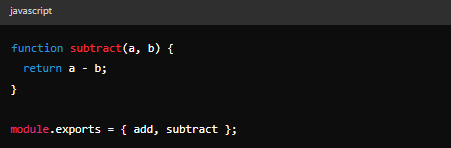
4.2 Write Tests for Subtract:
Update math.test.js
:
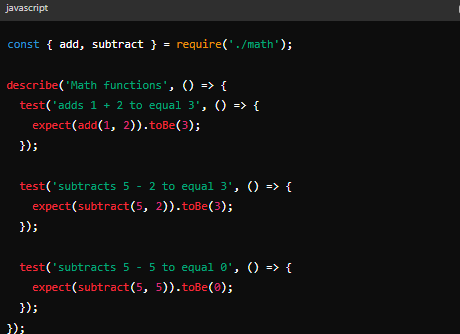
5. Mocking Dependencies
Sometimes, you need to test functions that depend on external modules or data. Jest provides tools to mock these dependencies.
5.1 Mocking a Module:
Suppose you have a function that fetches data from an API:
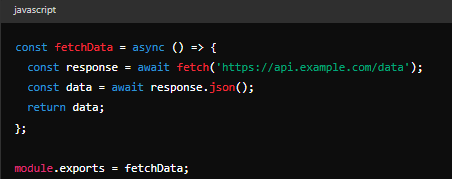
5.2 Mocking in Tests:
Create a test file fetchData.test.js
:
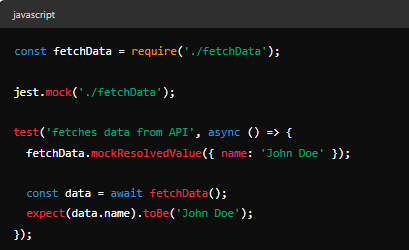
6. Best Practices for Writing Unit Tests
To write effective unit tests, keep the following best practices in mind:
6.1 Write Independent Tests: Each test should be independent and not rely on the state left by other tests.
6.2 Use Meaningful Test Names: Test names should clearly describe what the test is doing.
6.3 Test Edge Cases: Cover edge cases and potential failure scenarios.
6.4 Keep Tests Small and Focused: Each test should focus on a single aspect of the functionality.
Conclusion:
Writing effective unit tests in JavaScript is a fundamental skill for ensuring the reliability and maintainability of your codebase. By setting up a proper testing environment, organizing your tests, and following best practices, you can create robust tests that help catch bugs early and facilitate seamless development. Start integrating unit testing into your workflow and experience the benefits of having a well-tested codebase.